Building a Twitter bot to help thousands find COVID vaccines
During the early days of the COVID-19 vaccine rollout in 2021, finding an available appointment was like winning the lottery. Pharmacy websites would release batches of appointments sporadically, and they'd be gone within minutes. As a high school student with way too much free time during remote learning, I wondered if technology could help solve this problem.
The Problem
The vaccine distribution system wasn't prepared for the overwhelming demand. Pharmacies like CVS had digital systems in place, but they weren't built for:
- Millions of people checking simultaneously
- The need for real-time availability notifications
- The urgency of reaching vulnerable populations
Massachusetts residents were staying up all night refreshing pharmacy websites, joining Facebook groups to share tips, and spending hours navigating convoluted scheduling systems. There had to be a better way.
The Solution: @MassVax
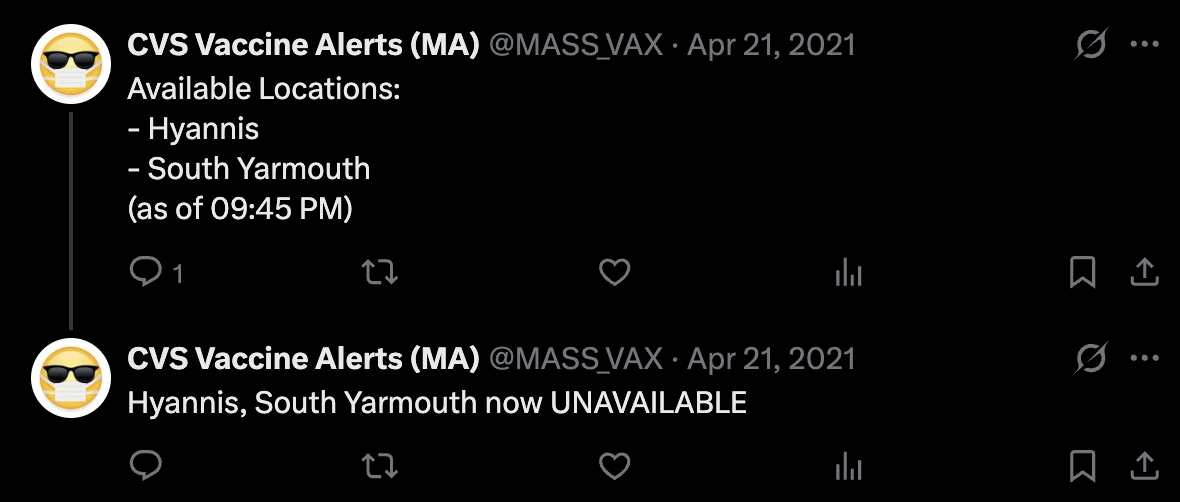
I built a Twitter bot that would continuously monitor CVS pharmacy websites for vaccine availability and instantly tweet whenever new appointments opened up. I called it @MassVax and it quickly gained traction.
Technical Implementation
The system architecture had a few key components:
1. Data Collection
I wrote a web scraper in Python that would check CVS's appointment system at regular intervals. This was trickier than it sounds - the website had anti-bot measures in place:
def rotate_identity(self):
current_agent = self.get_random_user_agent()
self.headers['user-agent'] = current_agent
current_proxy = self.get_random_proxy()
self.session.proxies = {
'http': f'http://{current_proxy}',
'https': f'https://{current_proxy}'
}
if random.random() < 0.2: # 20% chance: refresh cookies
self.session.cookies.clear()
I had to rotate user agents and IP addresses (via proxies) to avoid being blocked. The site also used cookies and other state mechanisms to detect automation, so I ended up simulating a real browser session using Selenium:
def simulate_human_form_completion(self):
screening_answers = {
"had_covid_recently": False,
"allergic_to_vaccine": False,
"health_conditions": False
}
for question, answer in screening_answers.items():
self.find_element(question)
time.sleep(random.uniform(0.5, 2.0)) # Human-like delay
self.select_option(question, answer)
time.sleep(random.uniform(1.0, 3.0))
self.click_button("Continue")
2. Data Processing
Once I could reliably collect data, I needed to determine when availability changed:
def detect_availability_changes(self):
new_locations = set(self.current_scan.available_locations)
previous_locations = set(self.previous_scan.available_locations)
# Find newly available locations
newly_available = new_locations - previous_locations
for location in newly_available:
self.logger.info(f"{location} is now AVAILABLE as of {self.timestamp}")
# Find locations no longer available
no_longer_available = previous_locations - new_locations
for location in no_longer_available:
self.logger.info(f"{location} is now UNAVAILABLE as of {self.timestamp}")
return bool(newly_available or no_longer_available)
This function compared the current list of available locations with the previous state and identified changes.
3. Twitter Integration
Using the Tweepy library, I set up automated tweets whenever new appointments became available:
def send_availability_updates(self, newly_available_locations):
timestamp = datetime.now().strftime("%I:%M %p")
if len(newly_available_locations) == 1: # Single location - simple tweet
location = list(newly_available_locations)[0]
message = f"Vaccine Available: {location} (as of {timestamp})"
tweet_id = self.twitter_api.post_tweet(message)
return tweet_id
elif len(newly_available_locations) > 1: # Multiple locations - create a thread
first_message = f"Multiple Vaccine Locations Available (as of {timestamp}):"
thread_starter_id = self.twitter_api.post_tweet(first_message)
location_batches = self._batch_locations(newly_available_locations)
for i, batch in enumerate(location_batches):
locations_text = "\n".join(f"• {loc}" for loc in batch)
reply = f"{locations_text}\n\n({i+1}/{len(location_batches)})"
self.twitter_api.post_reply(reply, thread_starter_id)
return thread_starter_id
For multiple locations, I created Twitter threads that listed all available appointment sites.
4. Reliability
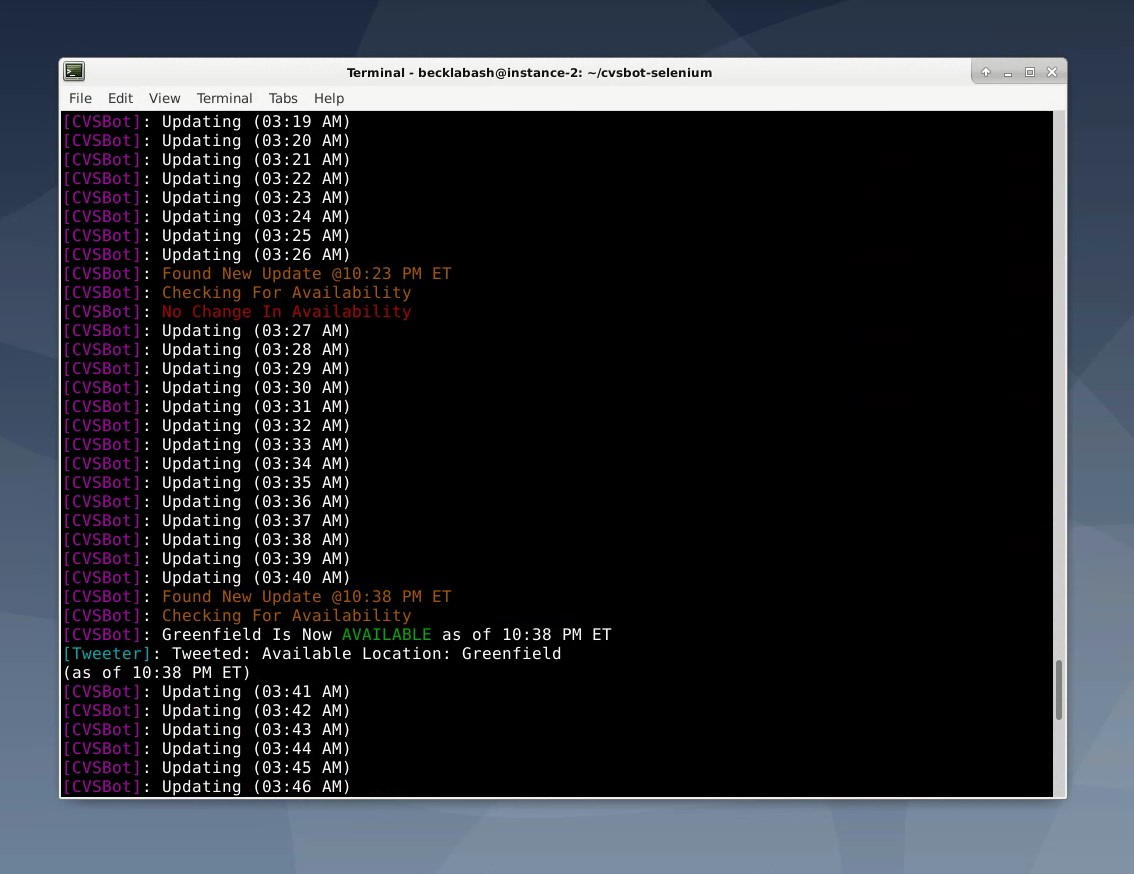
Since this was providing a critical service, I needed the bot to run 24/7 without interruption. I implemented:
- State saving between runs in case of crashes
- Logging for debugging issues
- Random delays between requests to appear more human-like
- Error handling for network issues
Impact
What started as a small personal project quickly grew beyond anything I had anticipated:
- The account reached nearly 10,000 followers at its peak
- I received messages from teachers and others in my community who successfully booked appointments for themselves or vulnerable family members
Technical Challenges
Building this wasn't without obstacles:
Challenge 1: The CVS Waiting Room
CVS implemented a virtual waiting room system that would sometimes activate during high traffic. This required special handling:
def detect_waiting_room_status(self):
"""
Check if the pharmacy website has activated its virtual waiting room.
Returns the current status and whether it has changed.
"""
try:
response = self.session.get(self.appointment_url, timeout=10)
if "waiting room" in response.text.lower() or "queue" in response.text.lower():
current_status = "WAITING_ROOM_ACTIVE"
elif "schedule appointment" in response.text.lower():
current_status = "DIRECT_ACCESS"
else:
current_status = "UNKNOWN"
status_changed = (current_status != self.previous_status)
self.previous_status = current_status
return current_status, status_changed
except Exception as e:
self.logger.error(f"Error checking waiting room: {str(e)}")
return "ERROR", False
Challenge 2: Avoiding Detection
The pharmacy websites were constantly updating their bot detection methods. I had to regularly adjust my approach, sometimes even implementing browser fingerprinting evasion techniques.
Challenge 3: Scale
As the account grew, I needed to ensure the system could handle increased scrutiny from both the pharmacy websites and Twitter's API rate limits.
Conclusion
As vaccine availability improved and the appointment crunch subsided, @MassVax became less necessary. I eventually retired the bot, but the experience of building something that tangibly helped people during a crisis has shaped how I think about technology and its potential for good.
The code isn't perfect - it was built quickly to address an urgent need - but it worked when people needed it most. Sometimes that's exactly what engineering is about: building practical solutions to real problems under time constraints.