The Problem
At Northeastern, students register for courses on a portal called Banner. After registration, the portal provides information about each course in a GUI. In order to access their course schedule through a calendar app (e.g. Google Calendar, Apple Calendar, Outlook), students must manually create events out of each course, including:
- Setting the correct recurring pattern (MWF vs TR)
- Adding location information
- Setting the time correctly
- Adding the course name and section
This is a tedious process, and it's error-prone.
The Solution
At Northeastern, courses are uniquely identified by a CRN. CRN2Cal is a React application that:
- Accepts CRNs as input
- Queries the university's course database API
- Generates calendar events in iCal (.ics) format
- Provides a downloadable file that can be imported into Google Calendar, Apple Calendar, Outlook, etc.
Through this process, CRN2Cal easily saves each student an hour per semester.
Technical Implementation
Frontend (React)
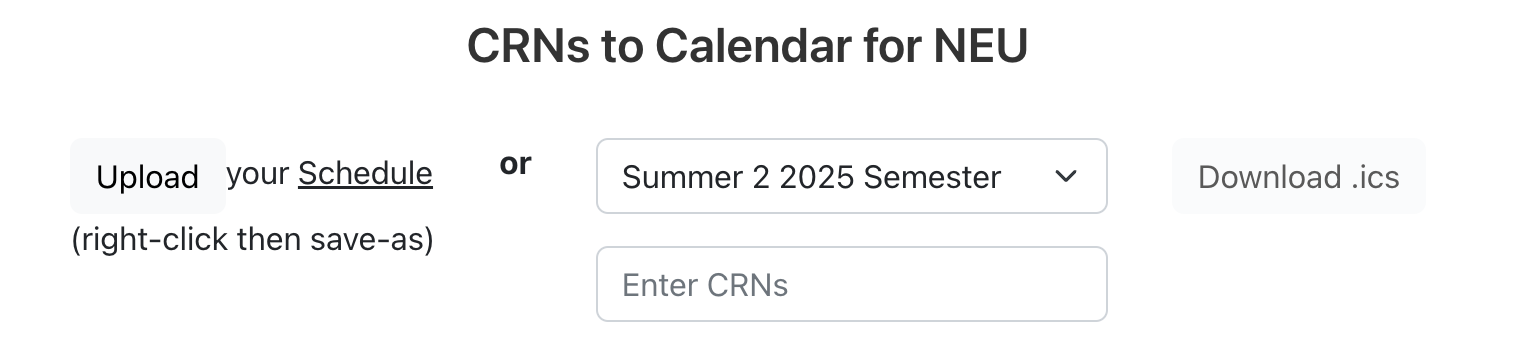
The user interface is built with React and styled with Bootstrap. It features:
- A simple form for manual CRN input
- An upload form that automatically parses CRNs from a student's
html
schedule page - Real-time validation to ensure provided CRNs exist in the Northeastern's course database
- Schedule download in iCal format
Course Data Retrieval
The application accesses the SearchNEU API to retrieve course information given a CRN. This is a student-developed service that already reverse-engineers the Banner API.
Calendar Generation
The core functionality uses the ics
library to generate the calendar events:
import { createEvents } from 'ics';
function generateCalendar(courses) {
const events = courses.map(course => ({
start: parseDateAndTime(course.startDate, course.startTime),
end: parseDateAndTime(course.startDate, course.endTime),
title: `${course.subject} ${course.courseNumber} - ${course.title}`,
description: `Section ${course.section}, CRN: ${course.crn}`,
location: course.location,
recurrenceRule: generateRecurrenceRule(course.daysOfWeek),
}));
return createEvents(events);
}
which can be imported into any calendar app that supports iCal format:
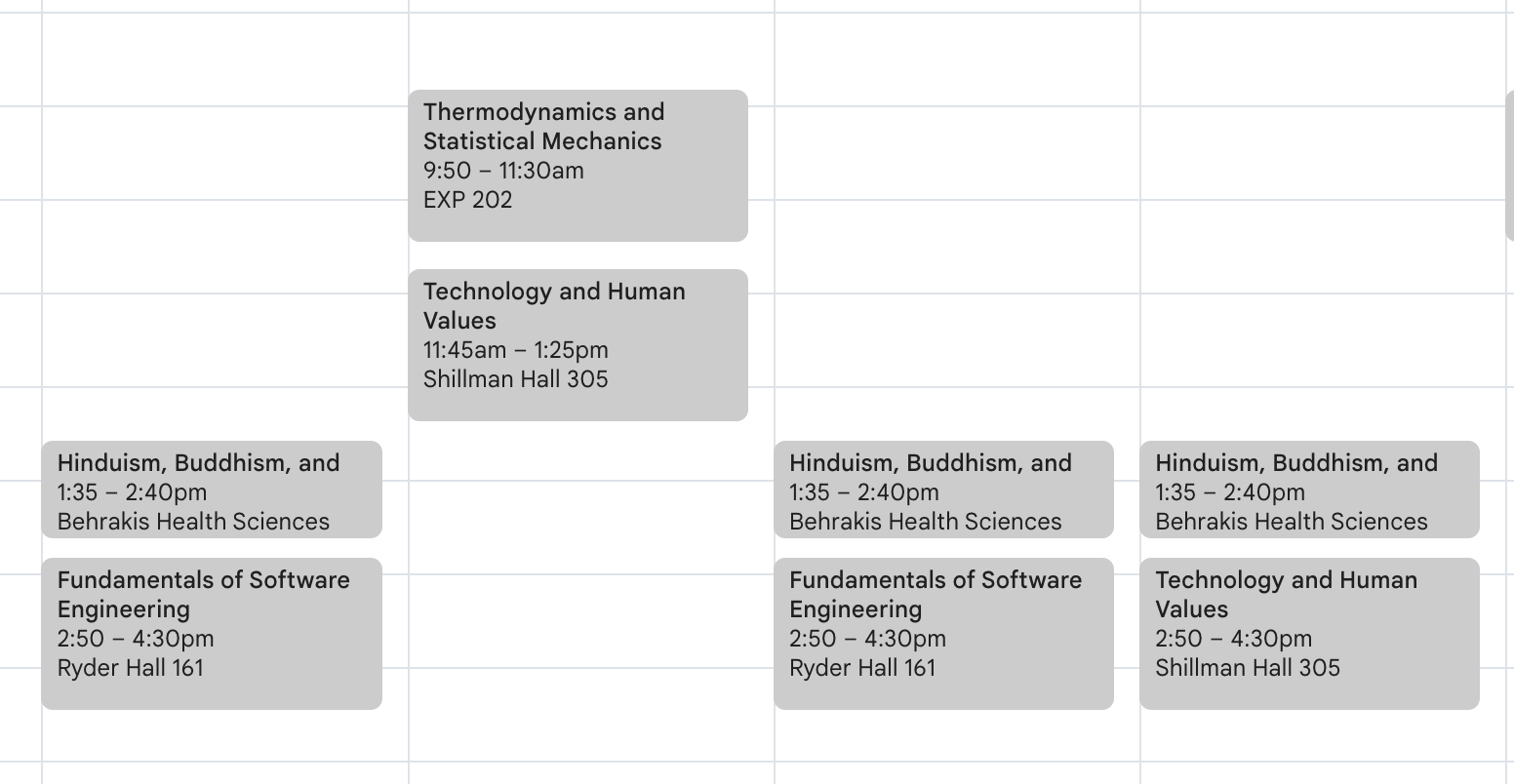